Android Development Roadmap: A Comprehensive Guide
- Date
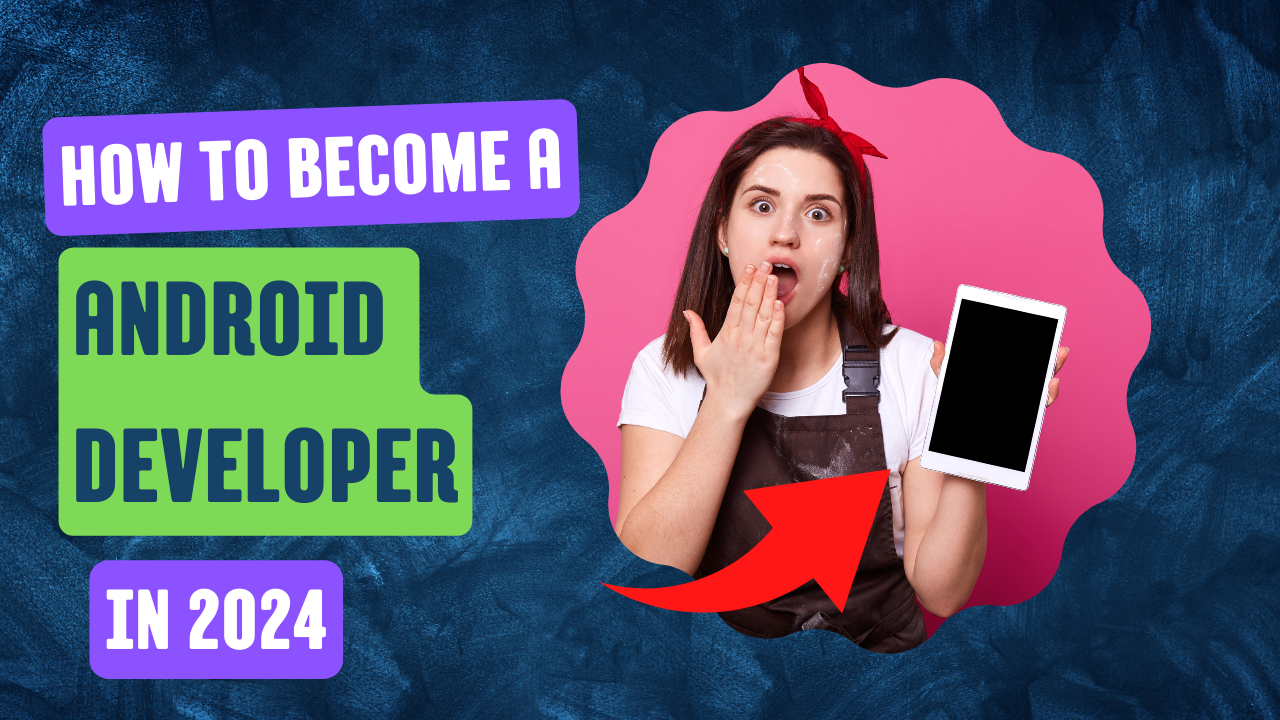
-
admin
Understanding the Basics
- Java or Kotlin: Choose one of these programming languages as the foundation for Android development. Kotlin is gaining popularity due to its modern features and interoperability with Java.
- Android Studio: This is the official IDE for Android development. It provides tools for building, testing, and debugging Android apps.
- Android SDK: The Software Development Kit contains tools, APIs, and libraries necessary for building Android apps.
Core Concepts
- Activities: The building blocks of Android apps. Each activity represents a single screen.
- Fragments: Reusable UI components that can be combined to create complex layouts within an activity.
- Layouts: Define the structure and appearance of your app's UI using XML.
- Intents: Messages that allow different components of your app to communicate.
- Services: Background tasks that run independently of the user interface.
- Broadcast Receivers: Respond to system-wide broadcasts, such as battery level changes or incoming calls.
UI Development
- Material Design: Adhere to Google's design guidelines to create visually appealing and consistent user interfaces.
- Custom Views: Create your own UI components to meet specific needs.
- Data Binding: Simplify UI development by automatically binding data to views.
App Architecture
- MVC (Model-View-Controller): Separate concerns into model (data), view (UI), and controller (logic).
- MVP (Model-View-Presenter): Improve testability by introducing a presenter layer.
- MVVM (Model-View-ViewModel): Use data binding and a ViewModel to simplify UI updates.
Testing
- Unit Tests: Test individual components of your app in isolation.
- UI Tests: Test the behavior of your app's user interface.
- Espresso: A popular framework for writing UI tests.
Performance Optimization
- Profiling: Identify performance bottlenecks using tools like Android Profiler.
- Memory Management: Avoid memory leaks and optimize memory usage.
- Battery Optimization: Minimize power consumption.
Advanced Topics
- Android Jetpack: A suite of libraries and tools that simplify common Android development tasks.
- Coroutines: Asynchronous programming made easy.
- Room: A persistence library for managing data in your app.
- WorkManager: Schedule background tasks that are resilient to device restarts.
Learning Resources
- Android Developer Documentation: Official documentation from Google.
- Coursera: Offers Android development courses.
- Udemy: Provides a variety of Android development courses.
- YouTube: Many tutorials and channels dedicated to Android development.
- Online communities: Join forums and communities to ask questions and learn from others.
Additional Tips
- Practice regularly: The more you code, the better you'll become.
- Stay updated: Android development is constantly evolving, so keep up with the latest trends and technologies.
- Build projects: Apply your knowledge by creating real-world Android apps.
- Contribute to open-source: Contribute to open-source projects to learn from others and give back to the community.
By following this roadmap and consistently practicing, you can become a proficient Android developer.