40 JavaScript Projects for Beginners – Easy Ideas to Get Started Coding JS
- Date
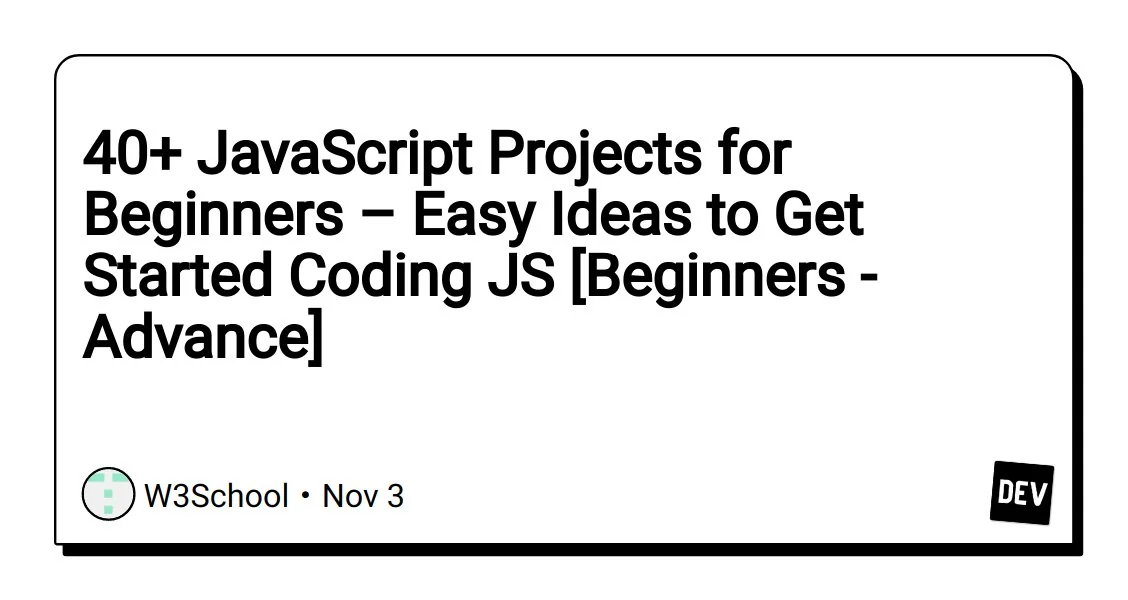
-
admin
Vanilla JavaScript Projects
If you have not learned JavaScript fundamentals, then I would suggest watching this course before proceeding with the projects.
Many of the screenshots below are from here.
1. How to create a Color Flipper

In this John Smilga tutorial, you will learn how to create a random background color changer. This is a good project to get you started working with the DOM.
In Leonardo Maldonado's article on why it is important to learn about the DOM, he states:
By manipulating the DOM, you have infinite possibilities. You can create applications that update the data of the page without needing a refresh. Also, you can create applications that are customizable by the user and then change the layout of the page without a refresh.
Key concepts covered:
arrays
document.getElementById()
document.querySelector()
addEventListener()
document.body.style.backgroundColor
Math.floor()
Math.random()
array.length
Before you get started, I would suggest watching the introduction where John goes over how to access the setup files for all of his projects.
How to create a Counter
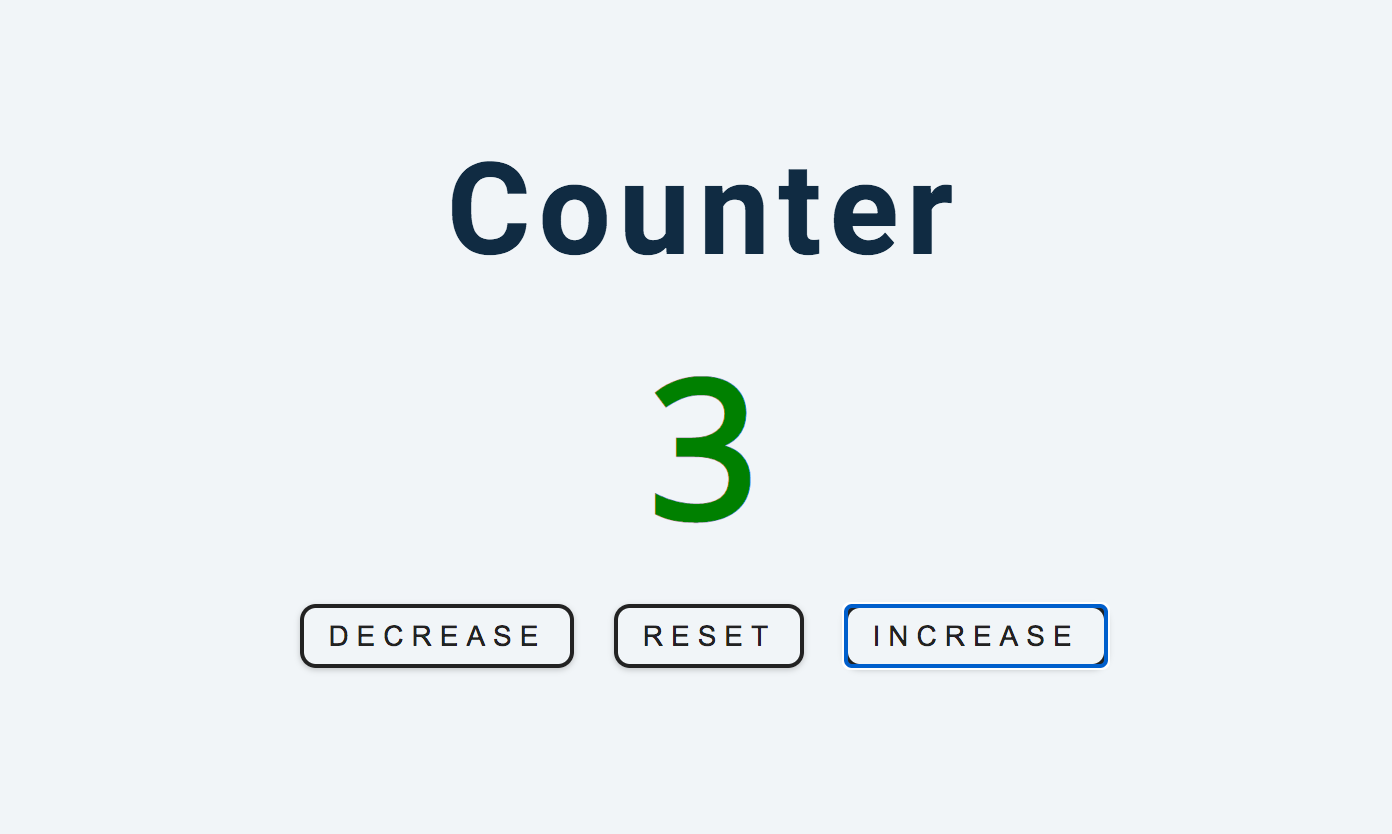
In this John Smilga tutorial, you will learn how to create a counter and write conditions that change the color based on positive or negative numbers displayed.
This project will give you more practice working with the DOM and you can use this simple counter in other projects like a pomodoro clock.
Key concepts covered:
document.querySelectorAll()
forEach()
addEventListener()
currentTarget property
classList
textContent
How to create a Review carousel

In this tutorial, you will learn how to create a carousel of reviews with a button that generates random reviews.
This is a good feature to have on an ecommerce site to display customer reviews or a personal portfolio to display client reviews.
Key concepts covered:
objects
DOMContentLoaded
addEventListener()
array.length
textContent
How to create a responsive Navbar
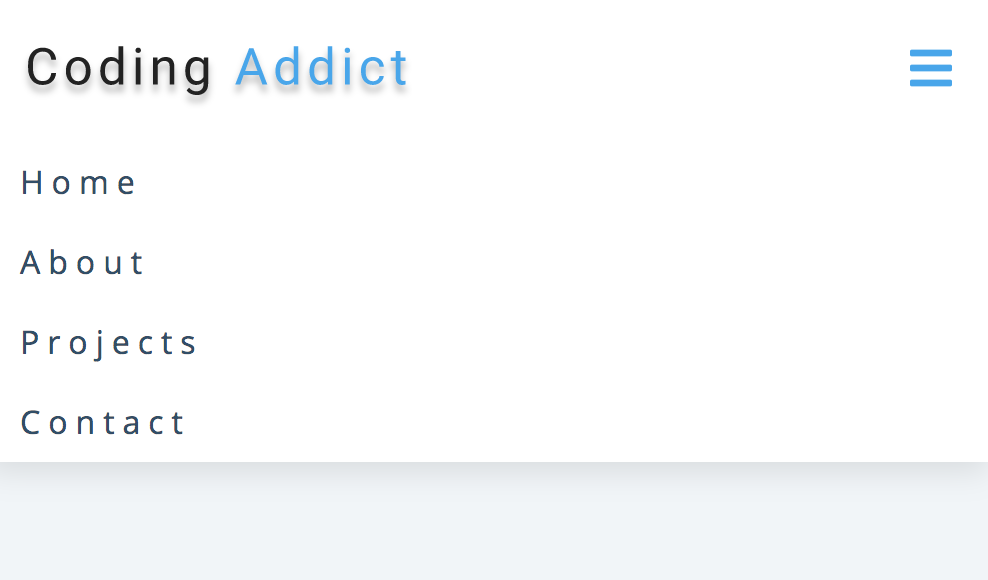
In this tutorial, you will learn how to create a responsive navbar that will show the hamburger menu for smaller devices.
Learning how to develop responsive websites is an important part of being a web developer. This is a popular feature used on a lot of websites.
Key concepts covered:
document.querySelector()
addEventListener()
classList.toggle()
How to create a Sidebar

In this tutorial, you will learn how to create a sidebar with animation.
This is a cool feature that you can add to your personal website.
Key concepts covered:
document.querySelector()
addEventListener()
classList.toggle()
classList.remove()
How to create a Modal
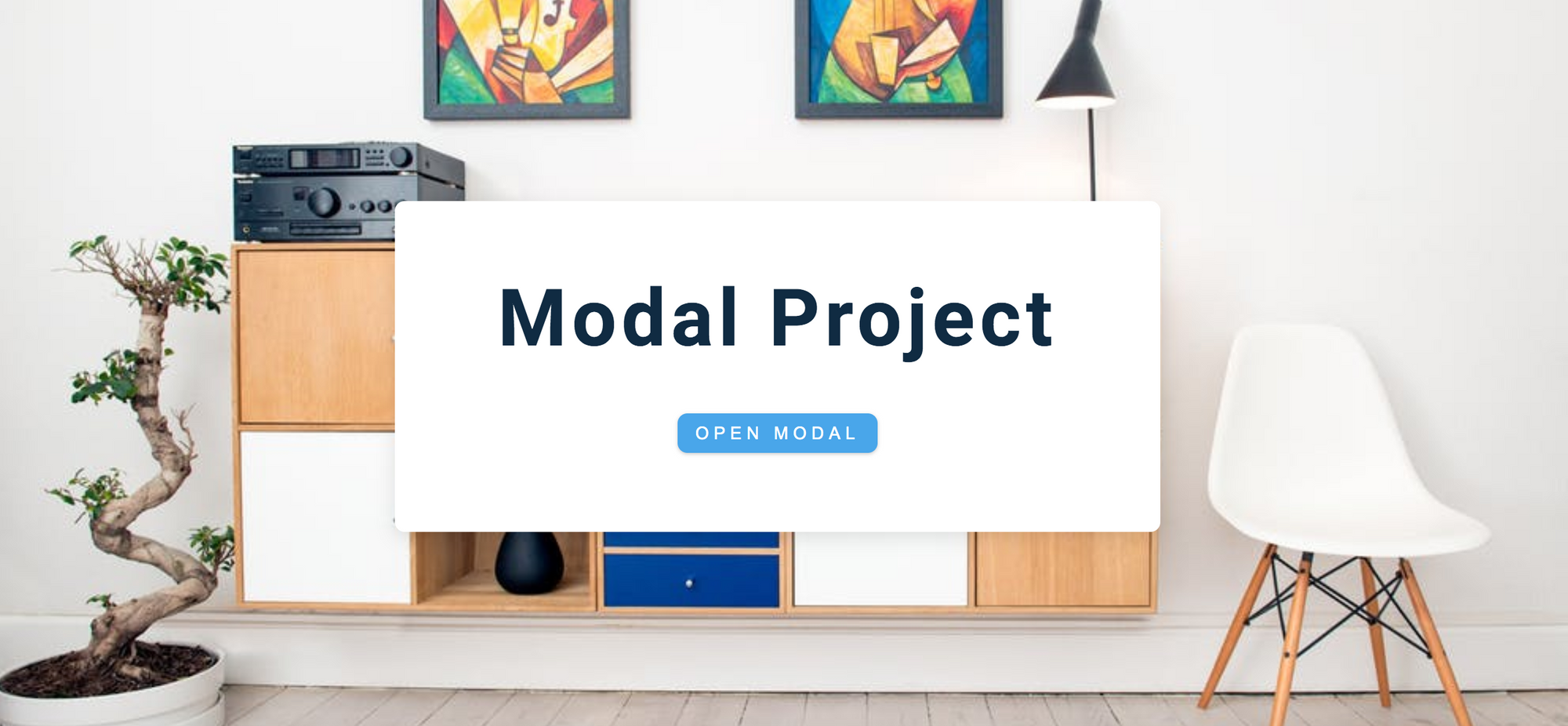
In this tutorial, you will learn how to create a modal window which is used on websites to get users to do or see something specific.
A good example of a modal window would be if a user made changes in a site without saving them and tried to go to another page. You can create a modal window that warns them to save their changes or else that information will be lost.
Key concepts covered:
document.querySelector()
addEventListener()
classList.add()
classList.remove()
How to create a FAQ page
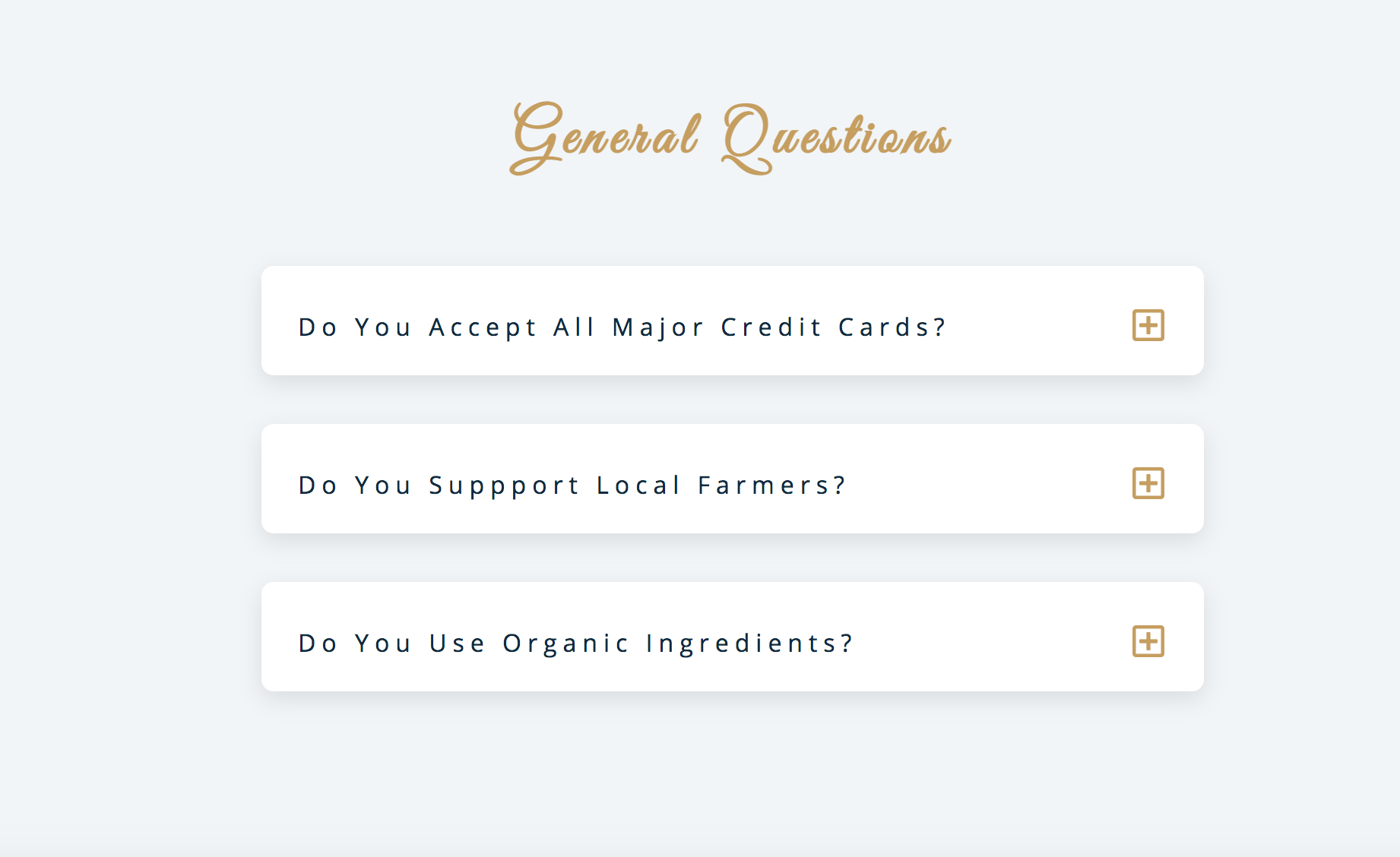
In this tutorial, you will learn how to create a frequently asked questions page which educates users about a business and drives traffic to the website through organic search results.
Key concepts covered:
document.querySelectorAll()
addEventListener()
forEach()
classList.remove()
classList.toggle()
How to create a restaurant menu page

In this tutorial, you will learn how to make a restaurant menu page that filters through the different food menus. This is a fun project that will teach you higher order functions like map, reduce, and filter.
In Yazeed Bzadough's article on higher order functions, he states:
the greatest benefit of HOFs is greater reusability.
Key concepts covered:
arrays
objects
forEach()
DOMContentLoaded
map, reduce, and filter
innerHTML
includes method
How to create a video background

In this tutorial, you will learn how to make a video background with a play and pause feature. This is a common feature found in a lot of websites.
Key concepts covered:
document.querySelector()
addEventListener()
classList.contains()
classList.add()
classList.remove()
play()
pause()
How to create a navigation bar on scroll
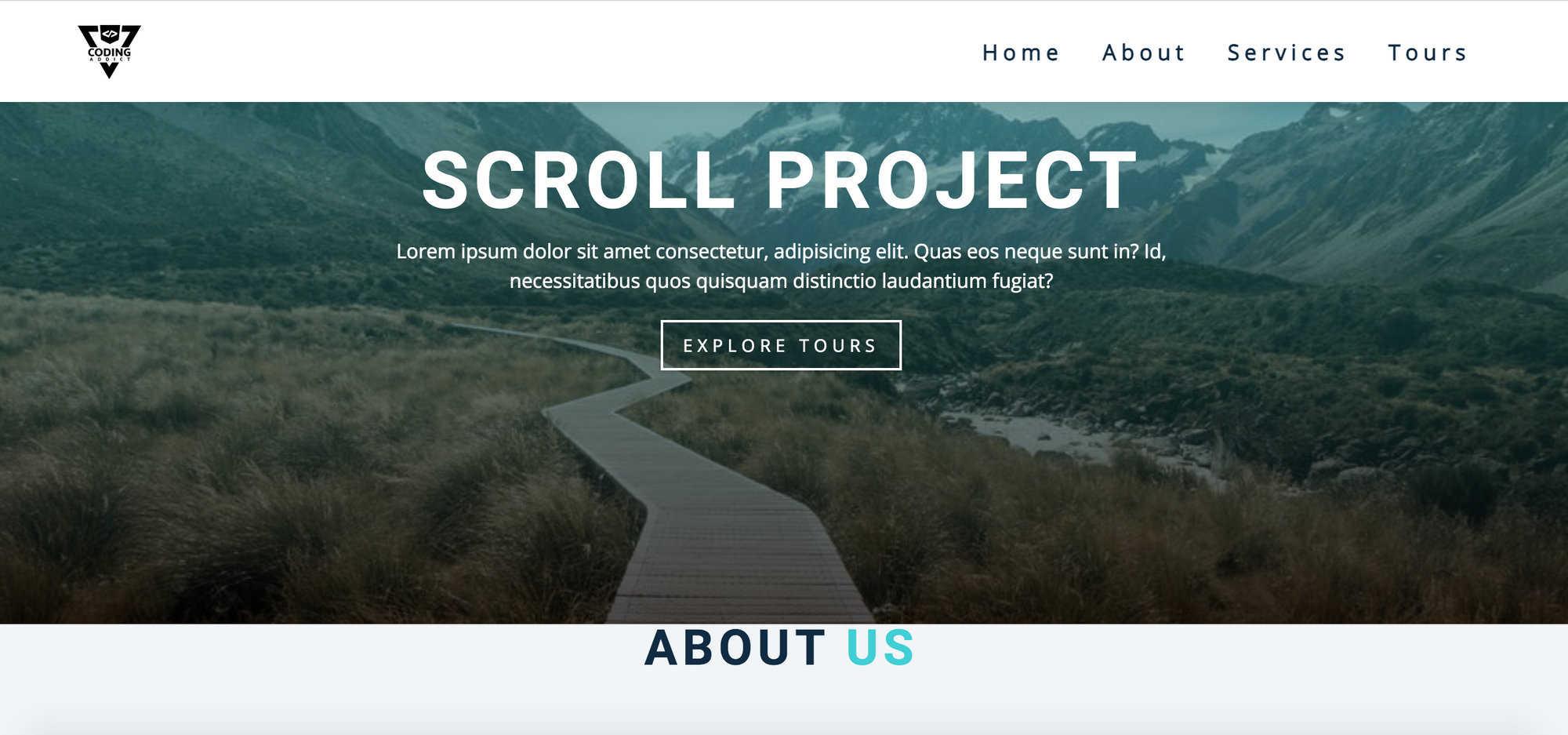
In this tutorial, you will learn how to create a navbar that slides down when scrolling and then stays at a fixed position at a certain height.
This is a popular feature found on many professional websites.
Key concepts covered:
document.getElementById()
getFullYear()
getBoundingClientRect()
slice method
window.scrollTo()
How to create tabs that display different content

In this tutorial, you will learn how to create tabs that will display different content which is useful when creating single page applications.
Key concepts covered:
classList.add()
classList.remove()
forEach()
addEventListener()
How to create a countdown clock
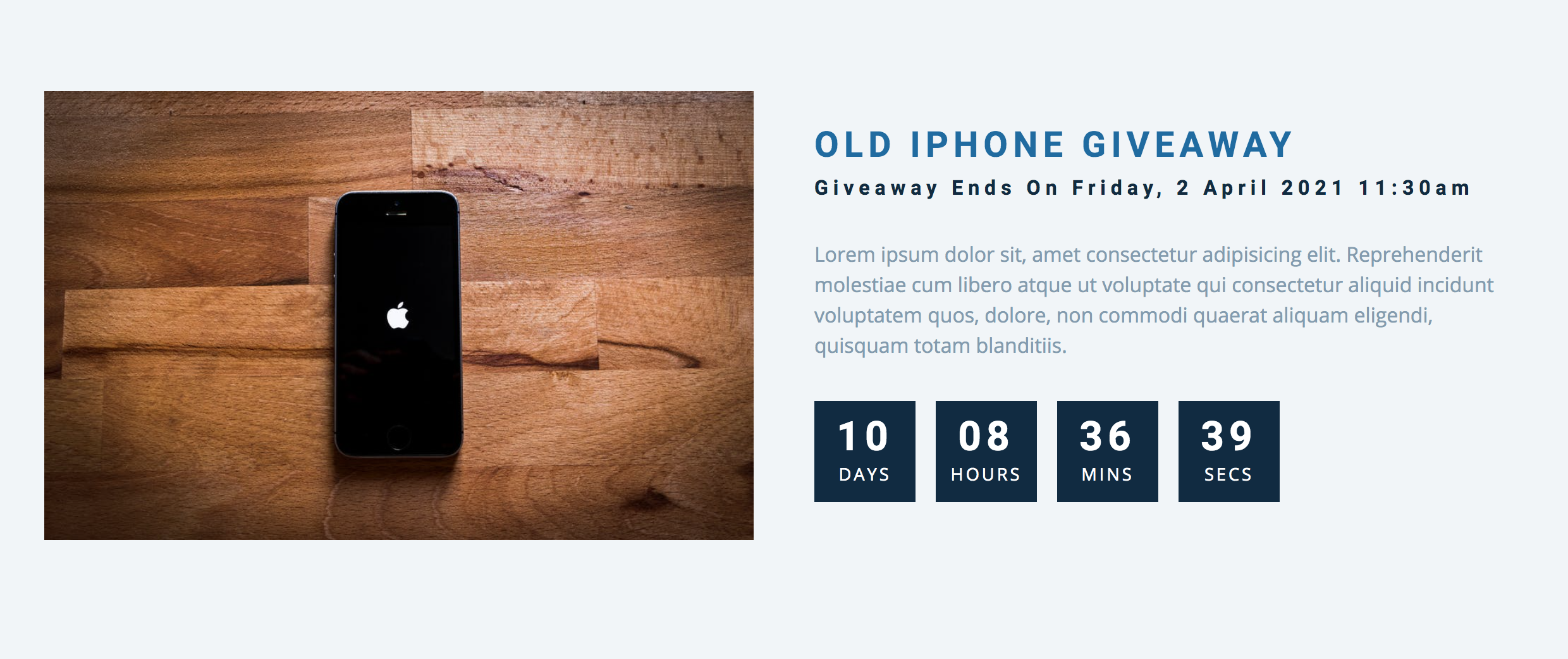
In this tutorial, you will learn how to make a countdown clock which can be used when a new product is coming out or a sale is about to end on an ecommerce site.
Key concepts covered:
getFullYear()
getMonth()
getDate()
Math.floor()
setInterval()
clearInterval()
How to create your own Lorem ipsum
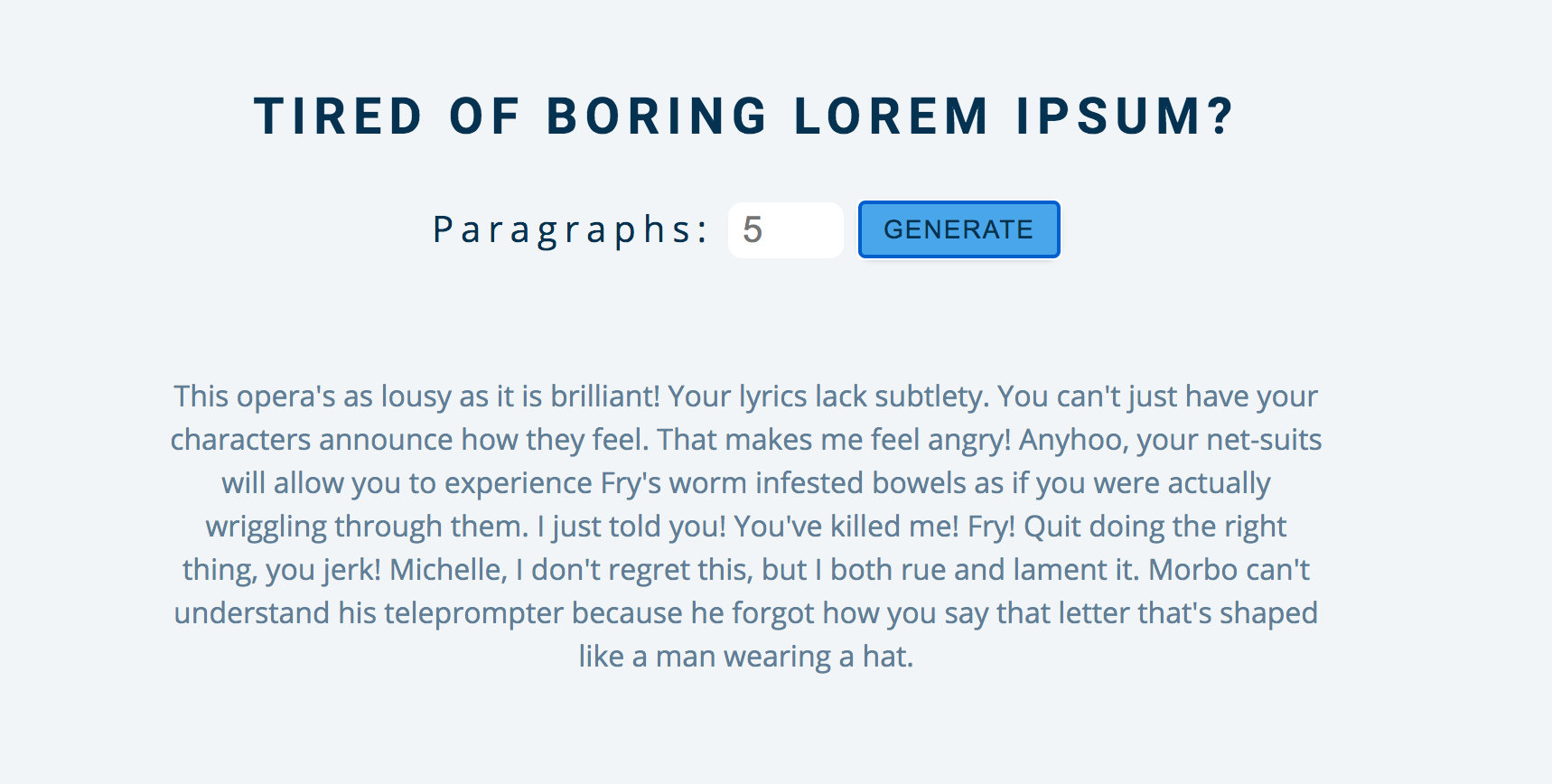
In this tutorial, you will learn how to create your own Lorem ipsum generator.
Lorem ipsum is the go to placeholder text for websites. This is a fun project to show off your creativity and create your own text.
Key concepts covered:
parseInt()
Math.floor()
Math.random()
isNaN()
slice method
event.preventDefault()
How to create a grocery list

In this tutorial, you will learn how to update and delete items from a grocery list and create a simple CRUD (Create, Read, Update, and Delete) application.
CRUD plays a very important role in developing full stack applications. Without it, you wouldn't be able to do things like edit or delete posts on your favorite social media platform.
Key concepts covered:
DOMContentLoaded
new Date()
createAttribute()
setAttributeNode()
appendChild()
filter()
map()
How to create an image slider
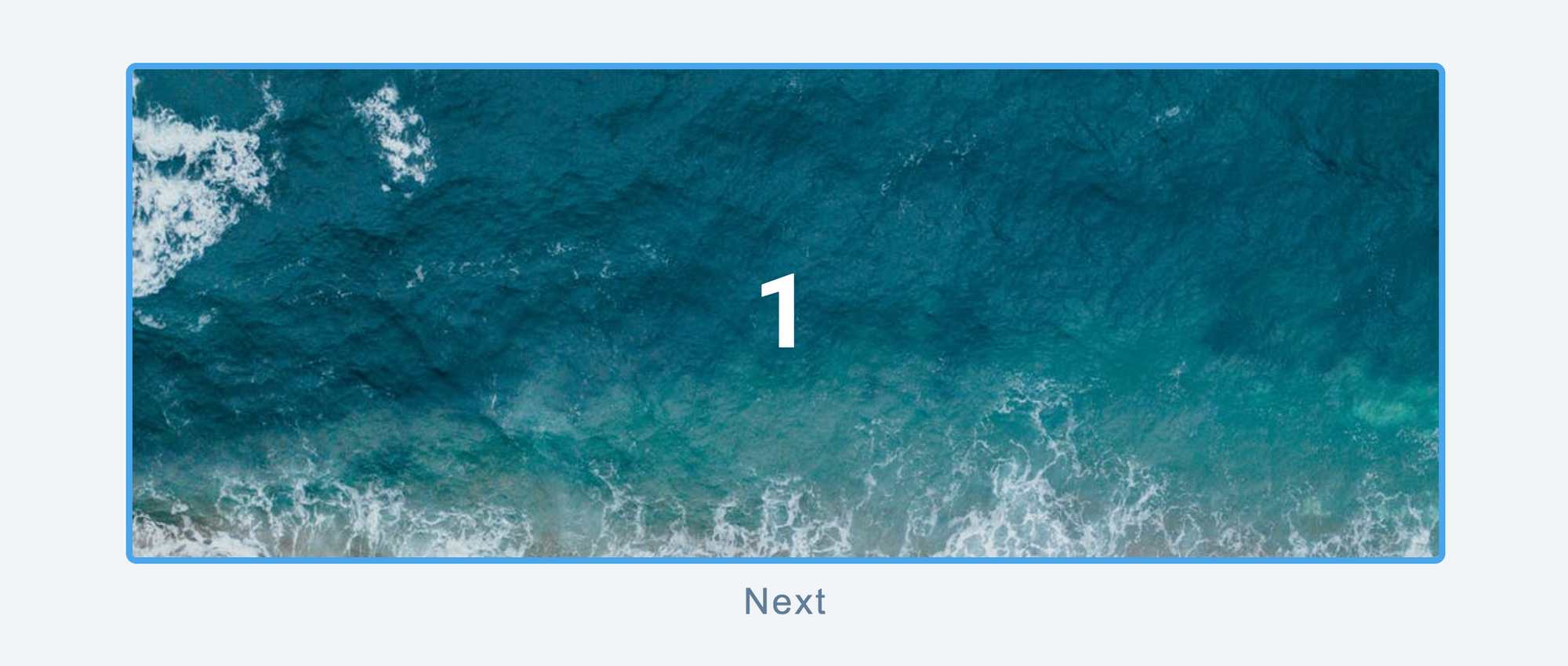
In this tutorial, you will learn how to build an image slider that you can add to any website.
Key concepts covered:
querySelectorAll()
addEventListener()
forEach()
if/else statements
How to create a Rock Paper Scissors game
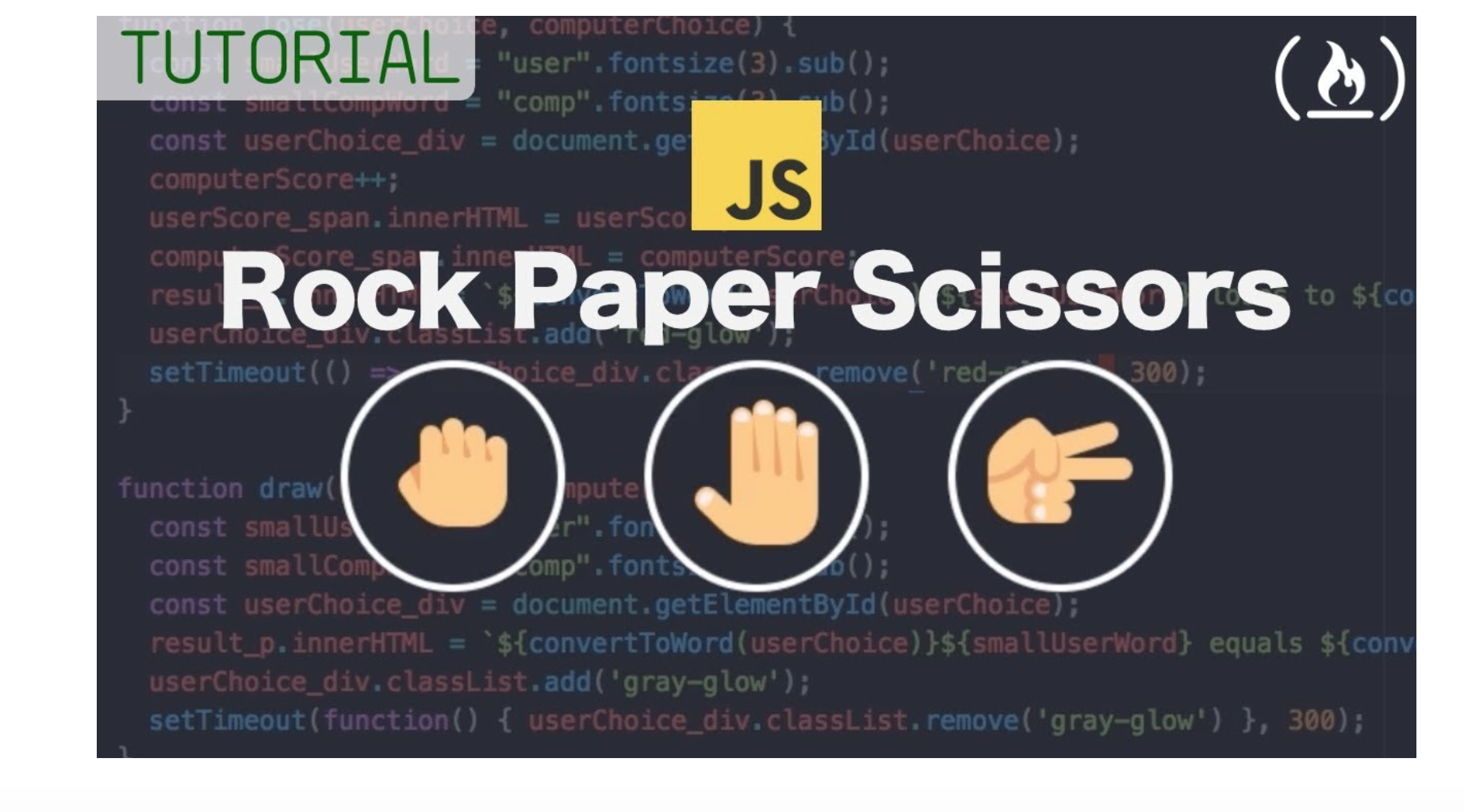
In this tutorial, Tenzin will teach you how to create a Rock Paper Scissors game. This is a fun project that will give more practice working with the DOM.
Key concepts covered:
addEventListener()
Math.floor()
Math.random()
switch statements
How to create a Simon Game

In this tutorial, Beau Carnes will teach you how to create the classic Simon Game. This is a good project that will get you thinking about the different components behind the game and how you would build out each of those functionalities.
Key concepts covered:
querySelector()
addEventListener()
setInterval()
clearInterval()
setTimeout()
play()
Math.floor()
Math.random()
How to create a Platformer Game
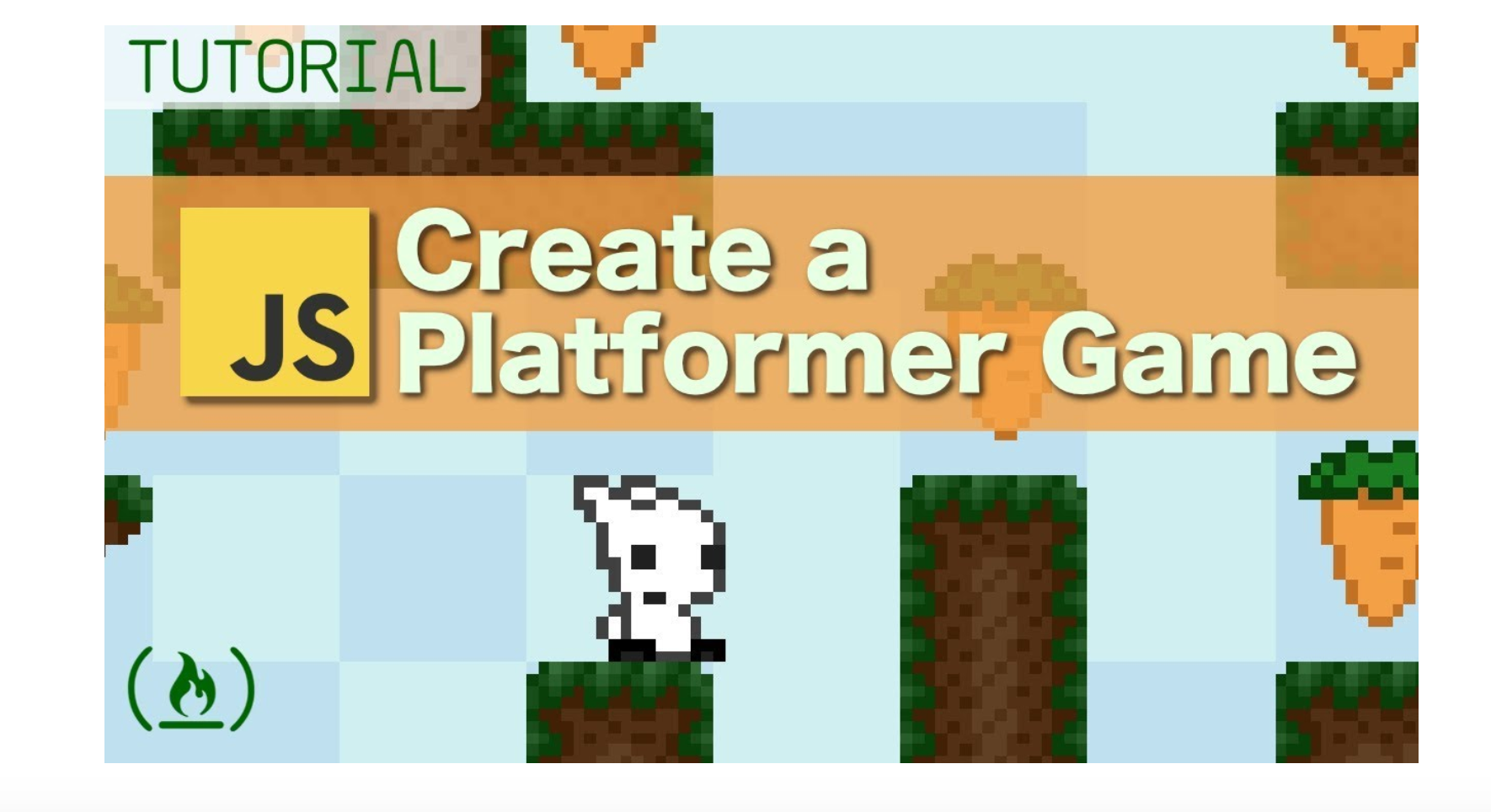
In this tutorial, Frank Poth will teach you how to build a platformer game. This project will introduce you to Object Oriented Programming principles and the Model, View, Controller software pattern.
Key concepts covered:
this keyword
for loop
switch statements
OOP principles
MVC pattern
Canvas API
How to create Doodle Jump and Flappy Bird

In this video series, Ania Kubow will teach you how to build Doodle Jump and Flappy Bird.
Building games are a fun way to learn more about JavaScript and will cover many popular JavaScript methods.
Key concepts covered:
createElement()
forEach()
setInterval()
clearInterval()
removeChild()
appendChild()
addEventListener()
removeEventListener()
How to create seven classic games with Ania Kubow

You will have a lot of fun creating seven games in this course by Ania Kubow:
Memory Game
Whack-a-mole
Connect Four
Snake
Space Invaders
Frogger
Tetris
Key concepts covered:
for loops
onclick event
arrow functions
sort()
pop()
unshift()
push()
indexOf()
includes()
splice()
concat()
React Projects
If you are not familiar with React fundamentals, then I would suggest taking this course before proceeding with the projects.
How to build a Tic-Tac-Toe game using React Hooks
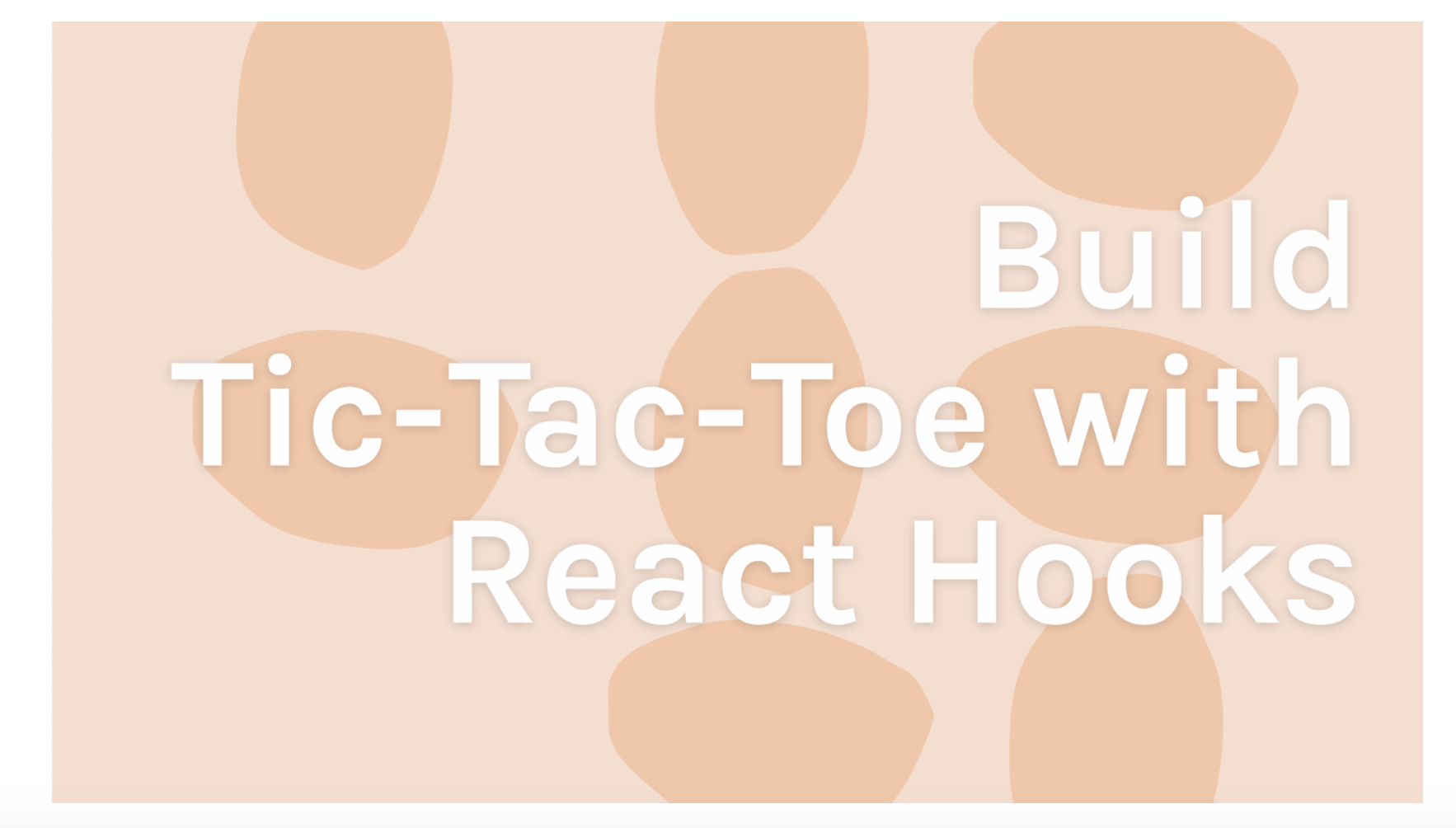
In this freeCodeCamp article, Per Harald Borgen talks about Scrimba's Tic-Tac-Toe game tutorial led by Thomas Weibenfalk. You can view the video course on Scimba's YouTube Channel.
This is a good project to start getting comfortable with React basics and working with hooks.
Key concepts covered:
useState()
import / export
JSX
How to build a Tetris Game using React Hooks
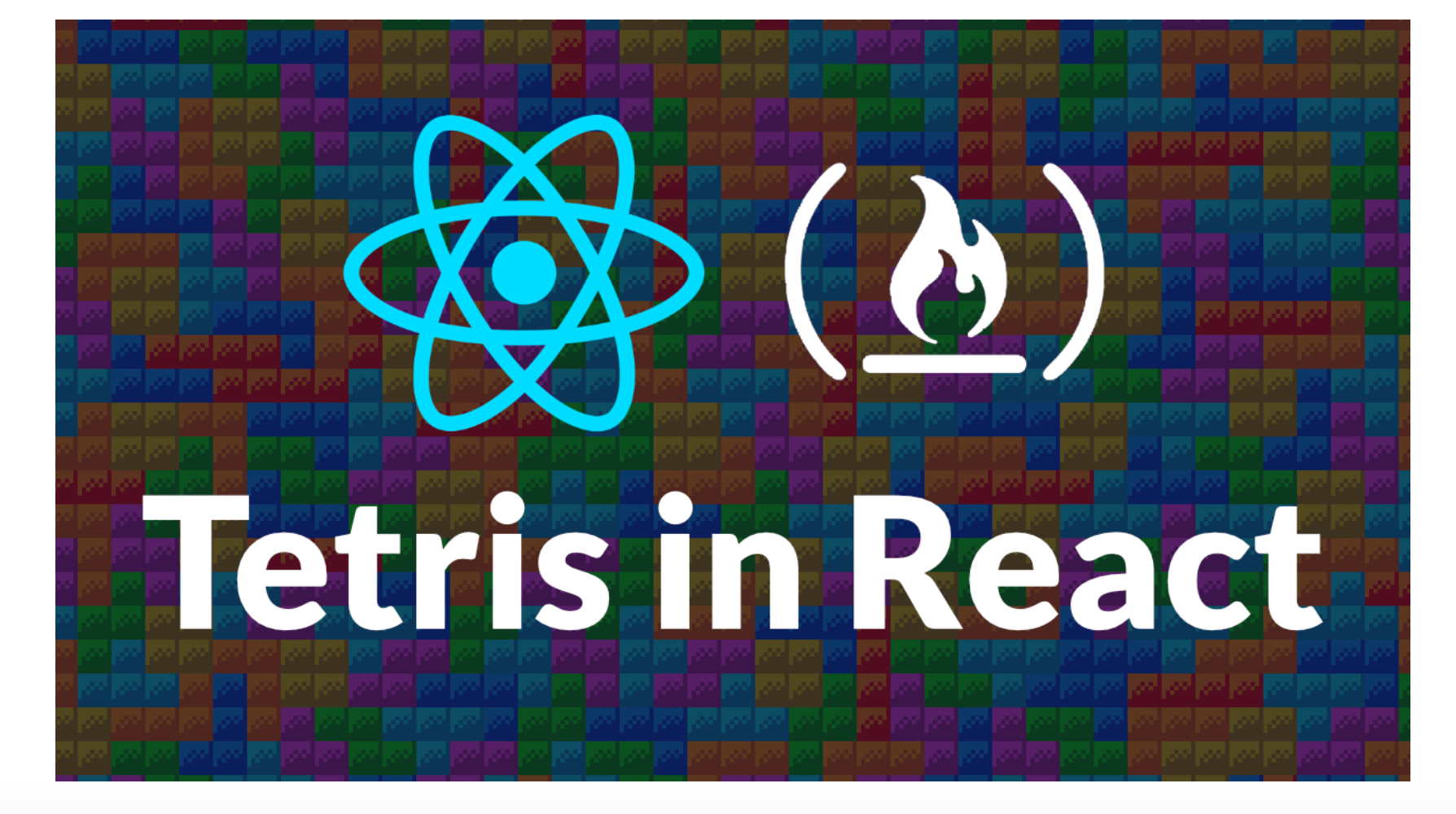
In this tutorial, Thomas Weibenfalk will teach you how to build a Tetris game using React Hooks and styled components.
Key concepts covered:
useState()
useEffect()
useRef()
useCallback()
styled components
How to create a Birthday Reminder App
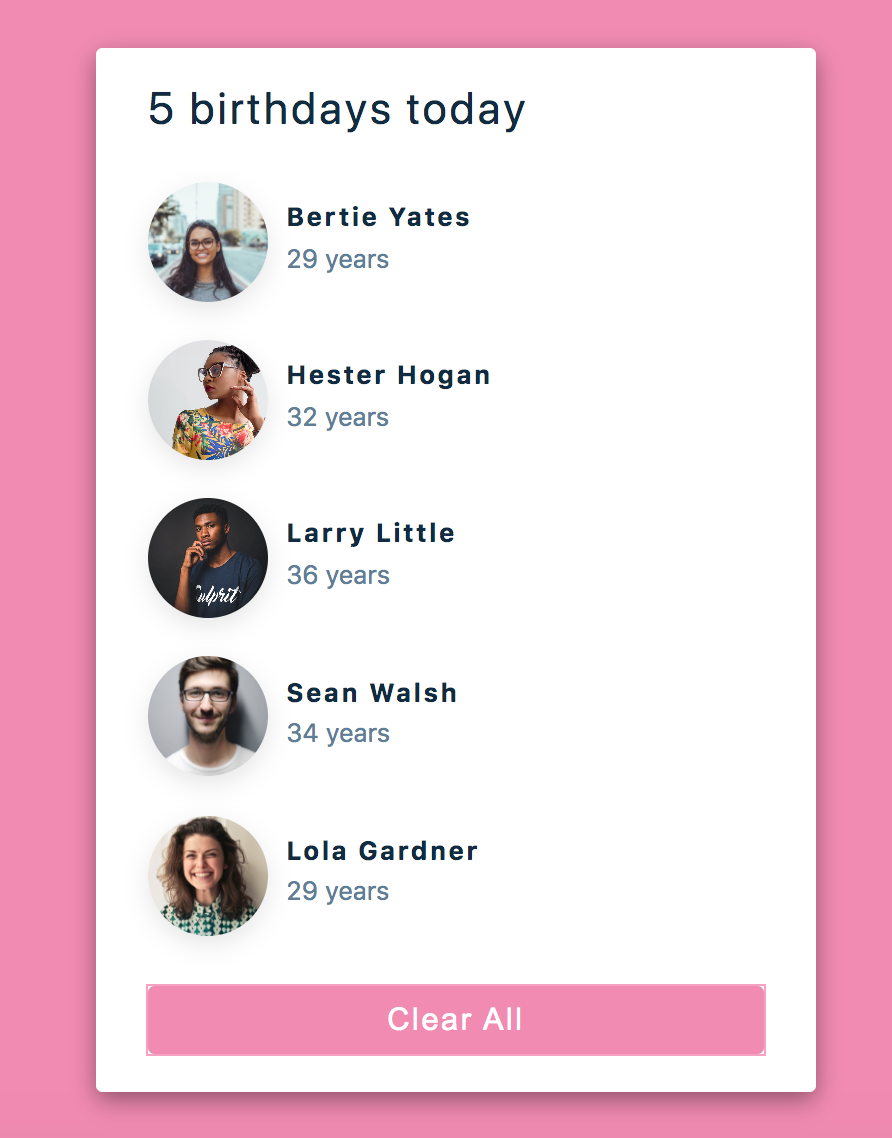
Screenshot from https://react-projects.netlify.app/
In this John Smilga course, you will learn how to create a birthday reminder app. This is a good project to start getting comfortable with React basics and working with hooks.
I would also suggest watching John's video on the startup files for this project.
Key concepts covered:
useState()
import / export
JSX
map()
How to create a Tours Page

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a tours page where the user can delete which tours they are not interested in.
This will give you practice with React hooks and the async/await pattern.
Key concepts covered:
try...catch statement
async/await pattern
useEffect()
useState()
How to create an accordion menu

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a questions and answers accordion menu. These menus can be helpful in revealing content to users in a progressive way.
Key concepts covered:
React icons
useState()
map()
How to create tabs for a portfolio page

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create tabs for a mock portfolio page. Tabs are useful when you want to display different content in single page applications.
Key concepts covered:
async/await pattern
React icons
useEffect()
useState()
How to create a review slider

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a review slider that changes to a new review every few seconds.
This is a cool feature that you can incorporate into an ecommerce site or portfolio.
Key concepts covered:
React icons
useEffect()
useState()
map()
How to create a color generator

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a color generator. This is a good project to continue practicing working with hooks and setTimeout.
Key concepts covered:
setTimeout()
clearTimeout()
useEffect()
useState()
try...catch statement
event.preventDefault()
How to create a Stripe payment menu page

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a Stripe payment menu page. This project will give you good practice on how to design a product landing page using React components.
Key concepts covered:
React icons
useRef()
useEffect()
useState()
useContext()
How to create a shopping cart page

Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a shopping cart page that updates and deletes items. This project will also be a good introduction to the useReducer hook.
Key concepts covered:
map()
filter()
<svg> elements
useReducer()
useContext()
How to create a cocktail search page
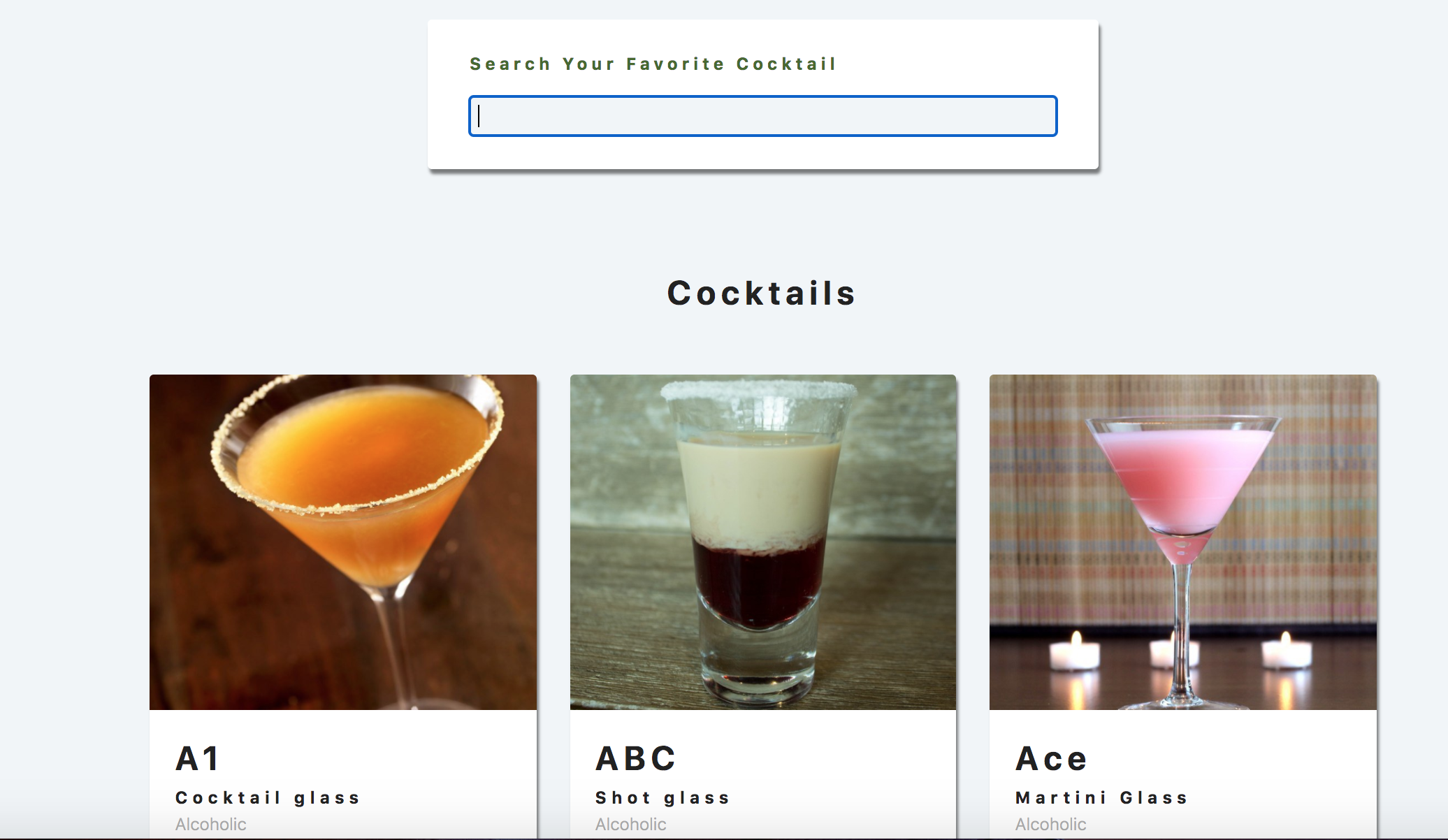
Screenshot from https://react-projects.netlify.app/
In this tutorial, you will learn how to create a cocktail search page. This project will give you an introduction to how to use React router.
React router gives you the ability to create a navigation on your website and change views to different components like an about or contact page.
Key concepts covered:
<Router>
<Switch>
useCallback()
useContext()
useEffect()
useState()
TypeScript Projects
If you are unfamiliar with TypeScript, then I would suggest watching this course before proceeding with this project.
How to build a Quiz App with React and TypeScript
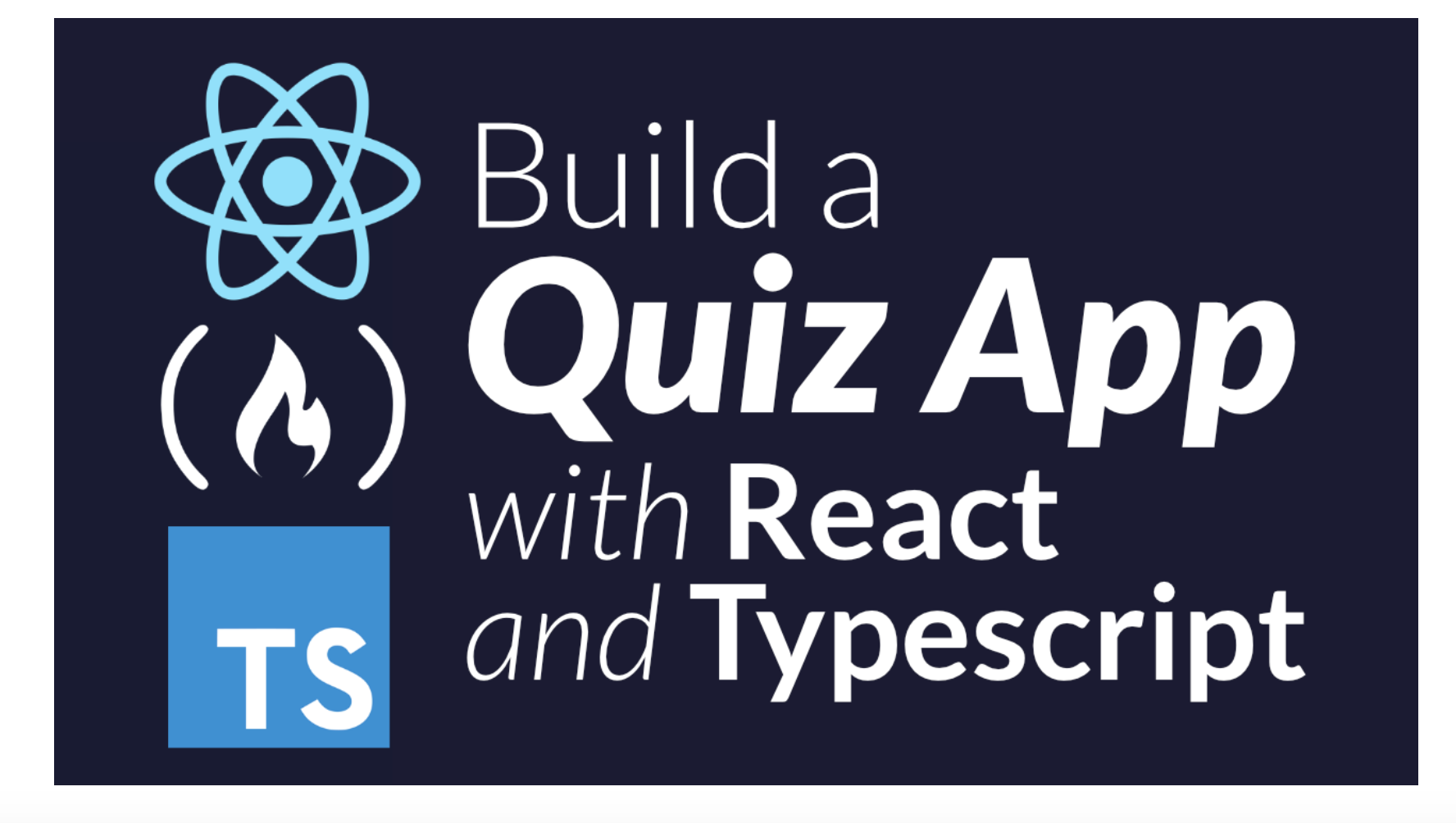
In this tutorial, Thomas Weibenfalk will teach you how to build a quiz app with React and TypeScript. This is a good opportunity to practice the basics of TypeScript.
Key concepts covered:
React.FC
styled components
dangerouslySetInnerHTML
How to create an Arkanoid game with TypeScript
